Learn What is JVM, JDK and JRE. The Java newbies often confuse between them and not sure whether to download a JRE or JDK. In this quick tutorial we will see what are the functions of these components, and the differences. This tutorial is part of an ongoing series of Java Tutorials.
Tutorial Contents
Overview
Before we actually go and see the details about JVM, JDK, and JRE we will have quick look at how a Java Program gets executed.

- A Java program is written in human readable language (.java)
- The program is compiled by
javac
(.class) - When the program is executed, an Execution Engine converts it to Machine Level instructions.
In this flow the JDK is responsible for compiling the Java Code. When the the program is executed JVM loads libraries, prepares JRE and transforms program to machine instructions.
Let’s have a look at all three of them in details.
Java Virtual Machine (JVM)
The Java Virtual Machine (JVM) is a heart of Java. You won’t see any option to Download a JVM anywhere because it is not shipped separately. But when you download a JDK or a JRE the JVM is always inside.
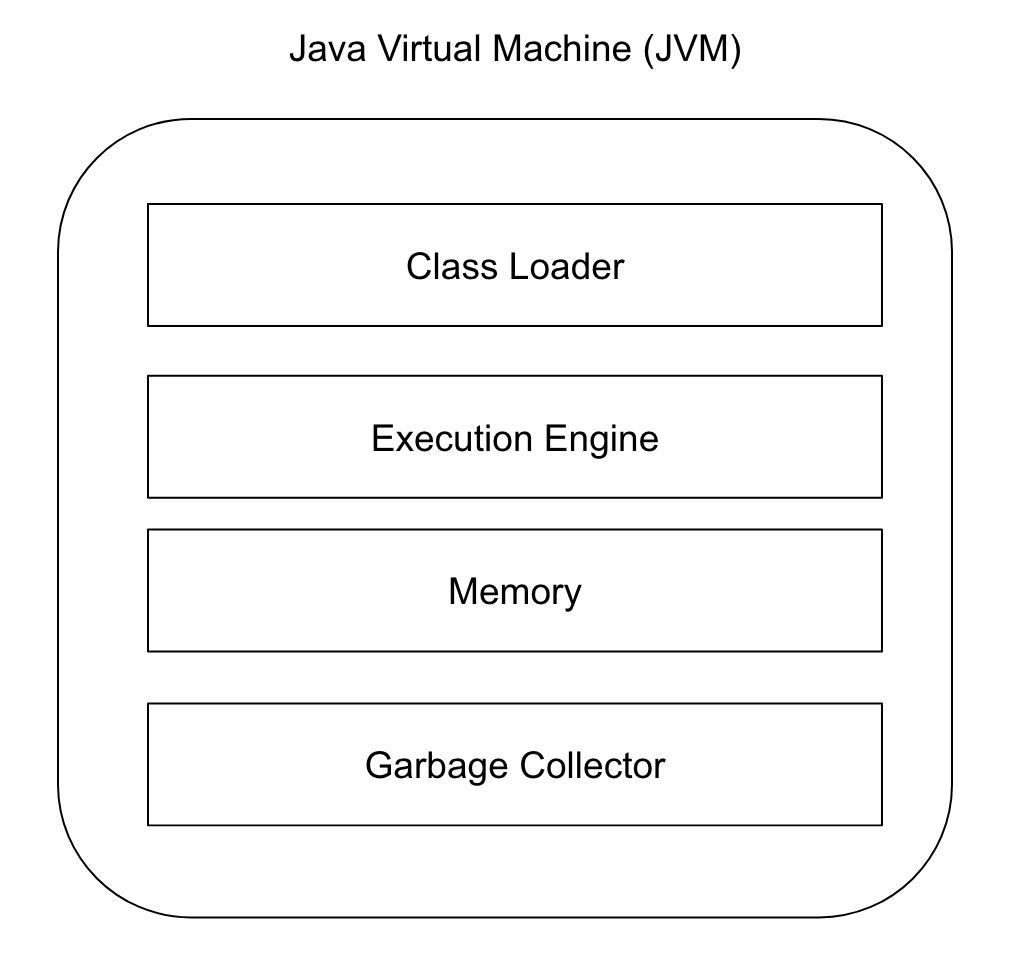
When we download Java we have to choose correct version based on our Operating System and the Processor. This is because the JVM is tightly coupled with Operating Systems. On the other hand JDK and JRE are absolutely the same across the Operating Systems and they can be downloaded once and used across different machines.
Other programming languages like C/C++ compile their codes directly into the machine level instructions. So each time you deploy your application you need to compile it on the particular system.
To avoid this Java never compiles the source code into machine instructions directly. It first convert the human readable source code into a generic bytecode. At runtime the JVM transforms the generic bytecode into the machine level instructions (Note: JVM is machine specific and it knows what instruction to prepare for a particular machine).
The Operating system comes into the picture only when the program is actually executed. This helps having a pre-compiled and packaged program prepared on one machine and transferred on another. This is the thing Java is well known for and it is called as “Write Once, Run Anywhere”.
The JVM is too broad topic to discuss and we will have a dedicated article for it.
Java Runtime Environment (JRE)
The Java Runtime Environment (JRE) is a software package that includes JVM, Libraries and Components required to run a Java Application. JRE is required when we try to run an application. Typically a deployment machine, for example production, will have JRE installed.
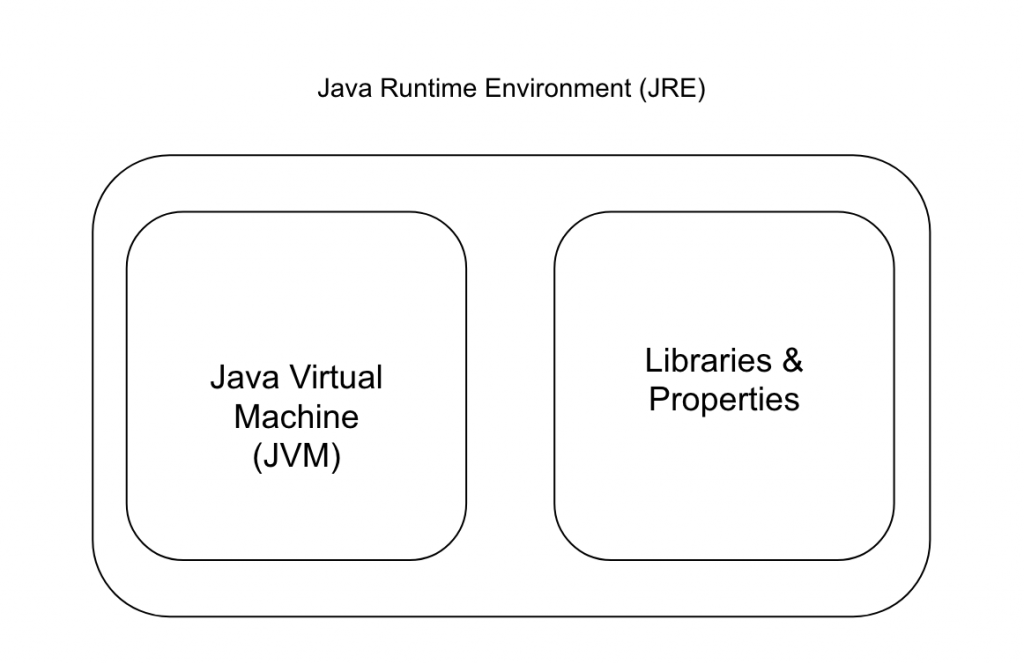
The JRE is consists of a Java Virtual Machine, Library classes, Property Files. Let’s have a look at them
Standard Library Classes
These are also called as Bootstrap classes. These are bunch of *.jar files under jre/lib
directory including rt.jar
, plugin.jar
, javaws.jar
. These jar contains Java’s standard Library classes.
What is rt.jar?
Java comes up with bunch of classes, interfaces and abstract classes like String, Map, Thread etc. All these classes are actually part of rt.jar. When you write or run a Java program the rt.jar should always be on the classpath.
Properties and Other Classes
Apart from the standard library classes, a JRE also has some extended library JARs and essential properties configurations. The properties contains configurations like deployment management and logging etc.
Java Development Kit (JDK)
The Java Development Kit (JDK) is a complete bundle of all the tools, binaries required for writing, Debugging, Compiling, Executing, Monitoring a Java Application. When we want to write and compile a Java Code we need to download JDK distribution.
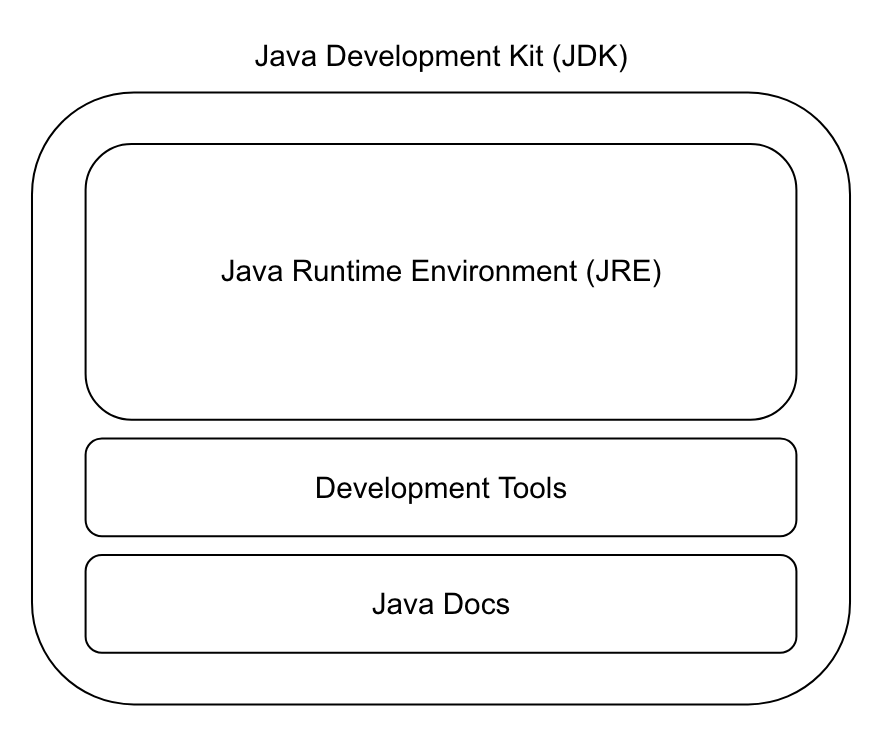
When you go and download a Java Development Kit, you will notice there are different versions for different Operating systems and processors. That is because JDK contains JVM and JVM is coupled with the Operating System.
As shown in the diagram JDK is a complete package of JRE + Java Docs + Development Tools.
Development Tools
Below are some of the development tools available in a JDK
- javac: This is a Java Compiler, required for compiling a Java Program
- java: Used to run a compiled Java application (this is also present in jre)
- javadoc: Used to generate java documentation.
- jar: Required to generate Java archives
- jconsole: Java memory and object management console.
Summary
In this article we learnt about the difference between Java Virtual Machine (JVM), Java Runtime Environment (JRE), and Java Development Kit (JDK). We saw that JVM is heart of Java which has basic tools to convert Java Program into Machine Instructions. The JRE is a package of JVM and Java Class Libraries. The JDK is a complete bundle required to develop and run Java application and it has JRE with few development tools