A Quick Guide to Spring Boot Auto Configuration. Learn the Spring Boot Feature with practical examples.
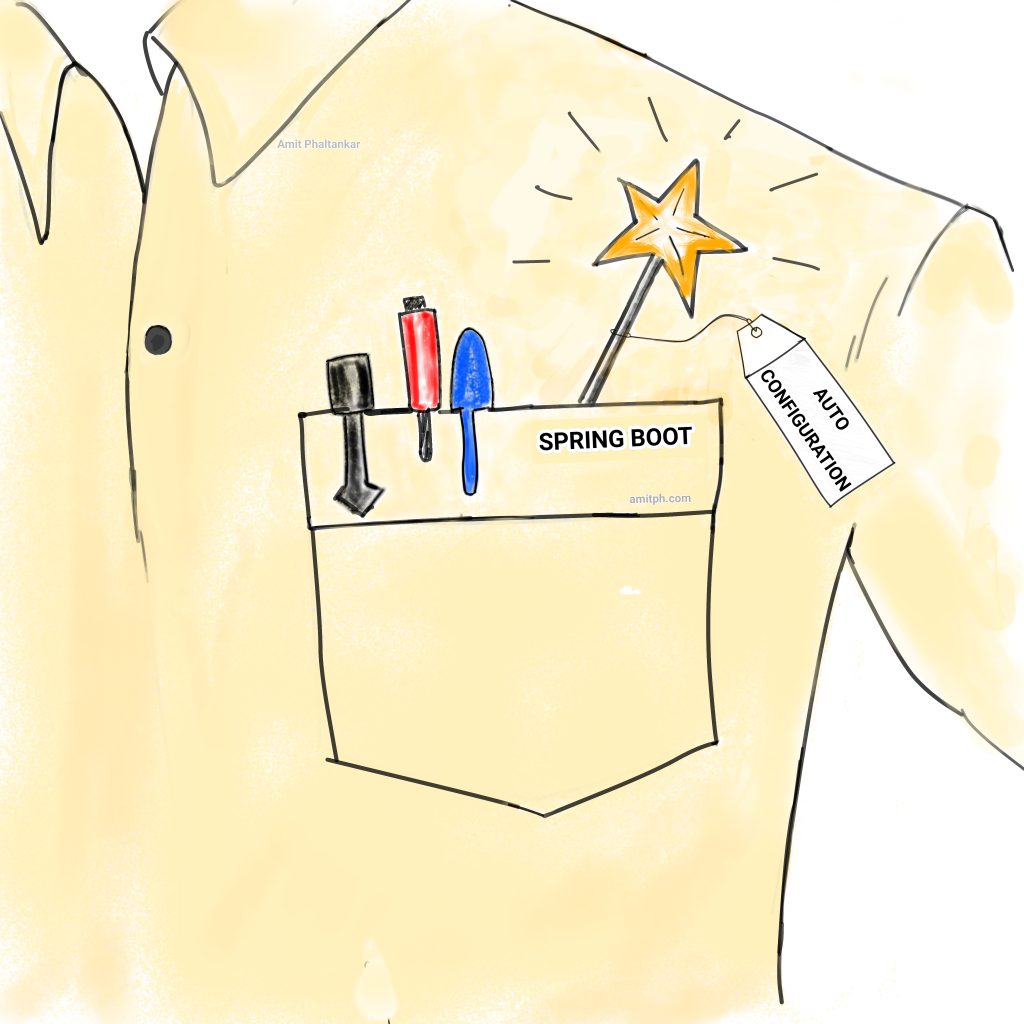
Auto Configuration is one of the most important features of Spring Boot and seems like some magic to many newbies. Having Auto configurations saves a lot of framework and component initialization fuss.
Tutorial Contents
What is Auto Configuration?
Auto Configuration is an ability of Spring Boot that automatically configures a Spring Application, analyzing the declared JAR dependencies.
Without Spring Boot
Consider a case where we want to use a spring application to query a database. We add a ‘spring-jdbc‘ dependency to the project and write XML configuration for creating a ‘datasource‘ and ‘jdbcTemplate‘.
Below is an extract of an actual Spring XML configuration which creates a datasource and a jdbcTemplate.
<!-- Create Datasource -->
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource" destroy-method="close">
<property name="driverClassName" value="${app.driverClassName}"/>
<property name="url" value="${app.url}"/>
<property name="username" value="${app.username}"/>
<property name="password" value="${app.password}"/>
</bean>
<!-- Load Properties -->
<context:property-placeholder location="app.properties"/>
<!-- Create JdbcTemplate -->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource"/>
</bean>
Code language: HTML, XML (xml)
The jdbcTemplate bean depends on the dataSource bean, which depends on various external configurations. Apart from the external variables, the bean configurations remain the same in every project we use the JdbcTemplate instance.
What if the instances were created in Java instead? – We still have repeating code, boilerplate code.
public DataSource getDataSource() {
DriverManagerDataSource dataSource =
new DriverManagerDataSource();
dataSource.setDriverClassName(driverClassName);
dataSource.setUrl(url);
dataSource.setUsername(dbUsername);
dataSource.setPassword(dbPassword);
return dataSource;
}
public JdbcTemplate getJdbcTemplate(){
return new JdbcTemplate(getDataSource());
}
Code language: Java (java)
Without Spring Boot, we write too much Boilerplate code.
Without Spring Boot, we have to add the same dependencies and initialize the same components with the same configurations. Spring Boot helps us avoid a repeating job in every project.
Learn more:
Benefits of Spring Boot Auto Configuration
Spring Boot understands our intentions from the libraries in the classpath. Like if we have added a dependency for JDBC, it is clear that our application wants to connect to a Database. It then uses its default set of configurations, loads all the required beans and their dependencies and makes them available for the application.
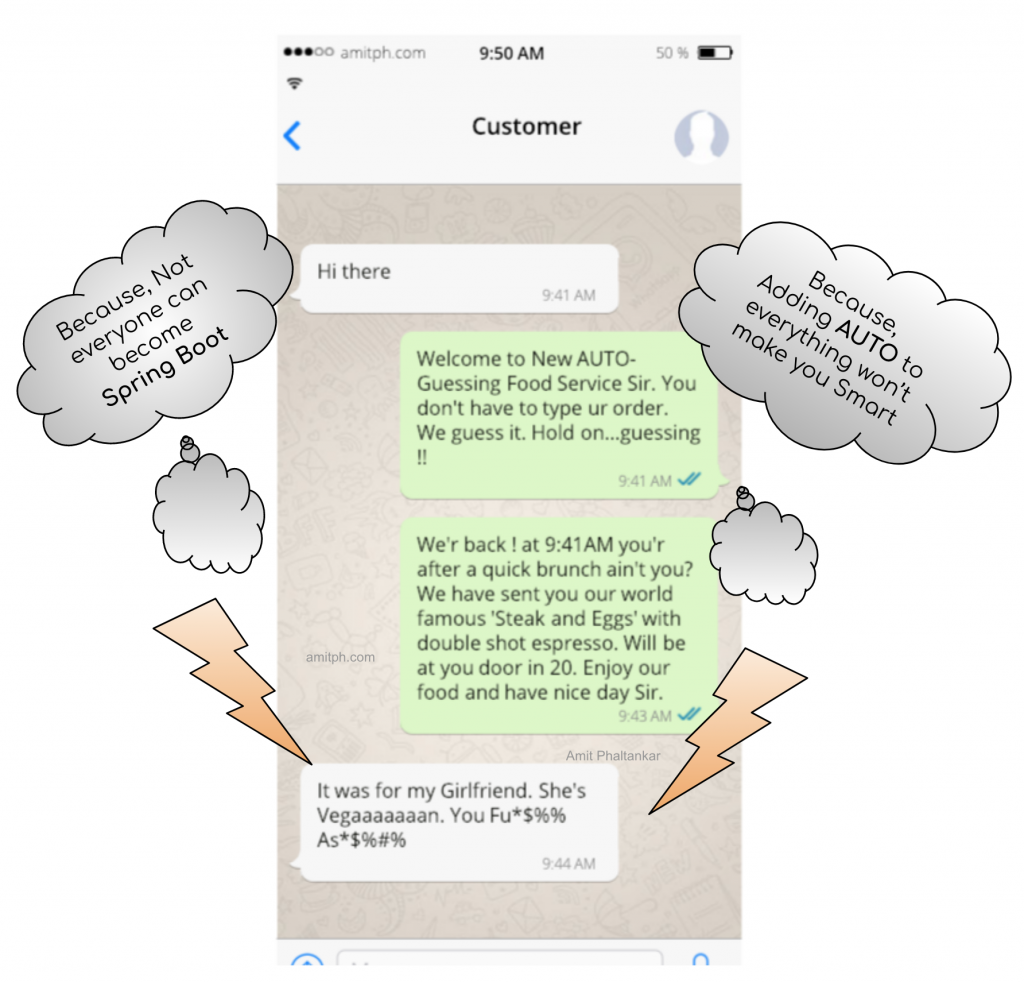
To achieve the auto-configuration as discussed, below are the things we need to do.
Starter Dependencies
Spring Boot Starters is a bunch of dependencies that frees us from managing transitive dependencies and their version compatibility. We add a particular starter dependency, for example, the ‘spring-boot-starter-jdbc‘, without any version. Spring Boot then downloads all related dependencies, including the components required to auto-configure the JDBC. Moreover, it also takes care of their version compatibility based on the current version of the Spring Boot.
Application Properties
Spring Boot provides a compelling and flexible means to manage application and environment-specific configurations. On top of that, Spring Boot supplies a predefined set of property keys and their default configurations that help us start the application with zero configurations. We can override the default configurations using environment-specific properties management.
@EnableAutoConfiguration
Then we will also need to tell Spring Boot that we want to rely on its auto-configuration feature. This is done by putting @EnableAutoConfiguration on our Application class or a @Configuration class. If we do not provide this annotation, Spring Boot assumes that we will initialize the components on our own.
Please note that the @EnableAutoConfigurationis included in the @SpringBootApplication annotation, and we don’t need to provide it explicitly.
Spring Boot Auto Configuration Example
It’s time for us to see the auto-configuration working.
Spring Boot Starter JDBC
Add Spring Boot Starter Jdbc Dependency.
For maven
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
Code language: HTML, XML (xml)
For Gradle
compile('org.springframework.boot:spring-boot-starter-jdbc')
Code language: Gradle (gradle)
Application Properties
spring:
datasource:
driverClassName: com.microsoft.sqlserver.jdbc.SQLServerDriver
password: '<password>'
url: jdbc:sqlserver://localhost:1433;databaseName=amitph-test-db
username: <username>
Code language: YAML (yaml)
Application Class
@SpringBootApplication
public class Application
implements CommandLineRunner {
@Autowired JdbcTemplate jdbcTemplate;
@Autowired DataSource dataSource;
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Override
public void run(String... args) throws Exception {
System.out.println("Autoconfigured JdbcTemplate = " + jdbcTemplate);
System.out.println("Autoconfigured Datasource = "+dataSource);
}
}
Code language: Java (java)
We can now run the application and see both jdbcTemplate and datasource are correctly assigned by Spring Boot.
Summary
Spring Boot Auto Configuration is a powerful feature of Spring Boot. It saves us from writing boilerplate code required to initialize certain components. The starter dependencies and the minimal properties help Spring Boot know which components to auto-configure.
2 thoughts on “Spring Boot Auto Configuration”
Very nice and useful
Thank you Bhauji 🙂
Comments are closed.